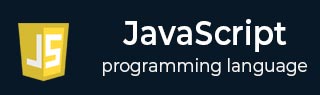
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript Number toExponential() Method
The JavaScript Number toExponential() method returns a string representing this number in exponential notation. If the optional parameter 'fractionDigits' is not in the range of [0, 100], this method throws a 'RangeError' exception. Additionally, if you try to invoke this method on an object that is not a number, you will get a 'TypeError' exception.
The fraction-Digits is an integer specifying the number of digits after the decimal point. Defaults to as many digits as necessary to specify the number.
The result of the toExponential() method is a string with the following format −
sign + '1.' + mantissa + 'e' + sign + exponent
Where,
- 'sign' is either + or - depeding on the sign of the number.
- 'mantissa' is the fractional part of the number, rounded to fractionDigits digtis.
- 'exponent' is the power of 10 that the number should be multiplied by
Syntax
Following is the syntax of JavaScript Number toExponential() method −
toExponential(fractionDigits)
Parameters
This method accepts an optional parameter called 'fractionDigits', which is described below −
- fractionDigits (optional) − An integer specifying the number of digits after the decimal point.
Return value
This method returns a string representing this number in exponential notation with one digit before the decimal point.
Example 1
In this example, we will demonstrate how to use the toExponential() method in JavaScript to represent a number in exponential notation.
<html> <head> <title>JavaScript toExponential() Method</title> </head> <body> <script> const val = 5; document.write("Given value = ", val); document.write("<br>Number in exponential notation = ", val.toExponential()); </script> </body> </html>
Output
The above program returns an exponential notation as "5e+0" −
Given value = 5 Number in exponential notation = 5e+0
Example 2
If we pass 3 as the value of an optional parameter (i.e. fractionDigits), this method will represent a number in exponential notation rounded to 3 decimal places.
<html> <head> <title>JavaScript toExponential() Method</title> </head> <body> <script> const val = 5.454312; const fractionDigits = 3; document.write("Given value = ", val); document.write("<br>Fraction digits value = ", fractionDigits); document.write("<br>Number in exponential notation = ", val.toExponential(fractionDigits)); </script> </body> </html>
Output
After executing the above program, it will return an exponential notation rounded to 3 decimal places as −
Given value = 5.454312 Fraction digits value = 3 Number in exponential notation = 5.454e+0
Example 3
If the value of the 'fractionDigits' parameter is outside the range of 0 to 100, this method will throw a "RangeError" exception.
<html> <head> <title>JavaScript toExponential() Method</title> </head> <body> <script> const val = 5.34565432; const fractionDigits = -1; document.write("Given value = ", val); document.write("<br> Fraction Digits value = ", fractionDigits); try { document.write("<br>Number in exponential notation = ", val.toExponential(-1)); } catch (error) { document.write("<br>",error); } </script> </body> </html>
Output
The above program throws a 'RangeError' exception.
Given value = 5.34565432 Fraction Digits value = -1 RangeError: toExponential() argument must be between 0 and 100
Example 4
The Number toExponential() method throws a "TypeError" exception if we invoke this method on an object that is not a number.
<html> <head> <title>JavaScript toExponential() Method</title> </head> <body> <script> const val = "abc"; const fractionDigits = 10; document.write("Given value = ", val); document.write("<br> Fraction Digits value = ", fractionDigits); try { document.write("<br>Number in exponential notation = ", val.toExponential(-1)); } catch (error) { document.write("<br>",error); } </script> </body> </html>
Output
The above program throws a 'TypeError' exception as shown below −
Given value = abc Fraction Digits value = 10 TypeError: val.toExponential is not a function
To Continue Learning Please Login