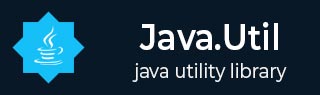
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java LinkedList clone() Method
Description
The Java LinkedList clone() returns a shallow copy of this LinkedList instance. This cloning ensures that there is no side-effect while copying and modifying the copy of linkedList.
Declaration
Following is the declaration for java.util.LinkedList.clone() method
public Object clone()
Parameters
NA
Return Value
This method returns a clone of this LinkedList instance.
Exception
NA
Cloning a LinkedList of Integer Example
The following example shows the usage of Java LinkedList clone() method. In this example, we're using Integers. At first, we're creating a linkedList as a new LinkedList object and then initialize with few items. As next step, we're cloning the linkedList1 to linkedList2 using clone() method call on linkedList1 object. In the end, we're printing linkedList2 to check if it contains copy of all elements of linkedList2 object.
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Integer> linkedList1 = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList1.add(1); linkedList1.add(2); linkedList1.add(3); linkedList1.add(4); // clone the first linkedList, LinkedList<Integer> linkedList2 = (LinkedList<Integer>)linkedList1.clone(); // let us print all the elements available in linkedList2 // now linkedList2 should have similar elements to linkedList1. System.out.println("LinkedList = " + linkedList2); } }
Output
Let us compile and run the above program, this will produce the following result −
LinkedList = [1, 2, 3, 4]
Cloning a LinkedList of String Example
The following example shows the usage of Java LinkedList clone() method. In this example, we're using Strings. At first, we're creating a linkedList as a new LinkedList object and then initialize with few items. As next step, we're cloning the linkedList1 to linkedList2 using clone() method call on linkedList1 object. In the end, we're printing linkedList2 to check if it contains copy of all elements of linkedList2 object.
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<String> linkedList1 = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList1.add("A"); linkedList1.add("B"); linkedList1.add("C"); linkedList1.add("D"); // clone the first linkedList, LinkedList<String> linkedList2 = (LinkedList<String>)linkedList1.clone(); // let us print all the elements available in linkedList2 // now linkedList2 should have similar elements to linkedList1. System.out.println("LinkedList = " + linkedList2); } }
Output
Let us compile and run the above program, this will produce the following result −
LinkedList = [A, B, C, D]
Cloning a LinkedList of Object Example
The following example shows the usage of Java LinkedList clone() method. In this example, we're using Student objects. At first, we're creating a linkedList as a new LinkedList object and then initialize with few items. As next step, we're cloning the linkedList1 to linkedList2 using clone() method call on linkedList1 object. In the end, we're printing linkedList2 to check if it contains copy of all elements of linkedList2 object.
package com.tutorialspoint; import java.util.LinkedList; public class LinkedListDemo { public static void main(String[] args) { // create an empty linkedList LinkedList<Student> linkedList1 = new LinkedList<>(); // use add() method to add elements in the linkedList linkedList1.add(new Student(1, "Julie")); linkedList1.add(new Student(2, "Robert")); linkedList1.add(new Student(3, "Adam")); // clone the first linkedList, LinkedList<Student> linkedList2 = (LinkedList<Student>)linkedList1.clone(); // let us print all the elements available in linkedList2 // now linkedList2 should have similar elements to linkedList1. System.out.println("LinkedList = " + linkedList2); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result −
LinkedList = [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]]
To Continue Learning Please Login