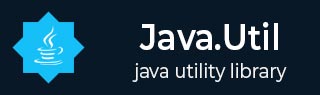
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java HashSet remove() Method
Description
The Java HashSet remove(Object o) method is used to remove the specified element from this set if it is present.
Declaration
Following is the declaration for java.util.HashSet.remove() method.
public boolean remove(Object o)
Parameters
o − This is the object to be removed from this set, if present.
Return Value
The method call returns 'true' if the set contained the specified element.
Exception
NA
Removing an Entry from the HashSet of Integers Example
The following example shows the usage of Java HashSet remove() method to remove an entry from the HashSet. We've created a HashSet object of Integer. Then few entries are added using add() method and then set is printed. Now set is updated by removing an entry using remove() method and printed again.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Integer> newset = new HashSet <>(); // populate hash set newset.add(1); newset.add(2); newset.add(3); // checking elements in hash set System.out.println("Hash set values: "+ newset); // remove 2 from the set newset.remove(2); // print the set System.out.println("Hash set values after remove operation: "+ newset); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set values: [1, 2, 3] Hash set values after remove operation: [1, 3]
Removing an Entry from the HashSet of Strings Example
The following example shows the usage of Java HashSet remove() method to remove an entry from the HashSet. We've created a HashSet object of String. Then few entries are added using add() method and then set is printed. Now set is updated by removing an entry using remove() method and printed again.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <String> newset = new HashSet <>(); // populate hash set newset.add("Learning"); newset.add("Easy"); newset.add("Simply"); // checking elements in hash set System.out.println("Hash set values: "+ newset); // remove "Easy" from the set newset.remove("Easy"); // print the set System.out.println("Hash set values after remove operation: "+ newset); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set values: [Learning, Easy, Simply] Hash set values after remove operation: [Learning, Simply]
Removing an Entry from the HashSet of Objects Example
The following example shows the usage of Java HashSet remove() method to remove an entry from the HashSet. We've created a HashSet object of Student object. Then few entries are added using add() method and then set is printed. Now set is updated by removing an entry using remove() method and printed again.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Student> newset = new HashSet <>(); // populate hash set newset.add(new Student(1, "Julie")); newset.add(new Student(2, "Robert")); newset.add(new Student(3, "Adam")); // checking elements in hash set System.out.println("Hash set values: "+ newset); // remove Robert from the set newset.remove(new Student(2, "Robert")); // print the set System.out.println("Hash set values after remove operation: "+ newset); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { if(obj == null) return false; Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } @Override public int hashCode() { return rollNo + name.hashCode(); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set values: [[ 2, Robert ], [ 1, Julie ], [ 3, Adam ]] Hash set values after remove operation: [[ 1, Julie ], [ 3, Adam ]]
To Continue Learning Please Login