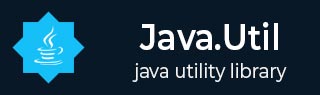
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java HashSet isEmpty() Method
Description
The Java HashSet isEmpty() method is used to check if this set contains no elements.
Declaration
Following is the declaration for java.util.HashSet.isEmpty() method.
public boolean isEmpty()
Parameters
NA
Return Value
The method call returns 'true' if this set contains no elements.
Exception
NA
Checking if HashSet of Integers is Empty or Not
The following example shows the usage of Java HashSet isEmpty() method to check if HashSet is empty or not. We've created a HashSet object of Integer. Then few entries are added using add() method and then set status is checked and printed using isEmpty() method. Now set is cleared using clear() method and then again set status is checked and printed using isEmpty() method.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Integer> newset = new HashSet <>(); // populate hash set newset.add(1); newset.add(2); newset.add(3); // checking elements in hash set System.out.println("Hash set is empty: "+ newset.isEmpty()); // clear the set newset.clear(); // print the set System.out.println("Hash set is empty after clearing: "+ newset.isEmpty()); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set is empty: false Hash set is empty after clearing: true
Checking if HashSet of Strings is Empty or Not
The following example shows the usage of Java HashSet isEmpty() method to check if HashSet is empty or not. We've created a HashSet object of String. Then few entries are added using add() method and then set status is checked and printed using isEmpty() method. Now set is cleared using clear() method and then again set status is checked and printed using isEmpty() method.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <String> newset = new HashSet <>(); // populate hash set newset.add("Learning"); newset.add("Easy"); newset.add("Simply"); // checking elements in hash set System.out.println("Hash set is empty: "+ newset.isEmpty()); // clear the set newset.clear(); // print the set System.out.println("Hash set is empty after clearing: "+ newset.isEmpty()); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set is empty: false Hash set is empty after clearing: true
Checking if HashSet of Objects is Empty or Not
The following example shows the usage of Java HashSet isEmpty() method to check if HashSet is empty or not. We've created a HashSet object of Student objects. Then few entries are added using add() method and then set status is checked and printed using isEmpty() method. Now set is cleared using clear() method and then again set status is checked and printed using isEmpty() method.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Student> newset = new HashSet <>(); // populate hash set newset.add(new Student(1, "Julie")); newset.add(new Student(2, "Robert")); newset.add(new Student(3, "Adam")); // checking elements in hash set System.out.println("Hash set is empty: "+ newset.isEmpty()); // clear the set newset.clear(); // print the set System.out.println("Hash set is empty after clearing: "+ newset.isEmpty()); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set is empty: false Hash set is empty after clearing: true
To Continue Learning Please Login