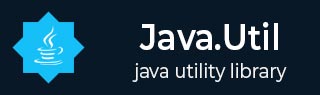
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java HashSet contains() Method
Description
The Java HashSet contains(Object o) method is used to return 'true' if this set contains the specified element.
Declaration
Following is the declaration for java.util.HashSet.contains() method.
public boolean contains(Object o)
Parameters
o − This is the element whose presence in this set is to be tested.
Return Value
The method call returns 'true' if this set contains the specified element.
Exception
NA
Checking if HashSet of Integers Contains an Entry or Not
The following example shows the usage of Java HashSet contains() method to check entries to be present in a HashSet. We've created a HashSet object of Integer. Then few entries are added using add() method and then set is printed. An existing entry is checked using contains() method and then a non-existing entry is checked and results are printed.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Integer> newset = new HashSet <>(); // populate hash set newset.add(1); newset.add(2); newset.add(3); // print the set System.out.println("Hash set: "+ newset); // checking elements in hash set System.out.println("Hash set contains 1: "+ newset.contains(1)); System.out.println("Hash set contains 4: "+ newset.contains(4)); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set: [1, 2, 3] Hash set contains 1: true Hash set contains 4: false
Checking if HashSet of Strings Contains an Entry or Not
The following example shows the usage of Java HashSet contains() method to check entries to be present in a HashSet. We've created a HashSet object of Strings. Then few entries are added using add() method and then set is printed. An existing entry is checked using contains() method and then a non-existing entry is checked and results are printed.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <String> newset = new HashSet <>(); // populate hash set newset.add("Learning"); newset.add("Easy"); newset.add("Simply"); // print the set System.out.println("Hash set: "+ newset); // checking elements in hash set System.out.println("Hash set contains - Easy: "+ newset.contains("Easy")); System.out.println("Hash set contains - Tutorials: "+ newset.contains("Tutorials")); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set: [Learning, Easy, Simply] Hash set contains - Easy: true Hash set contains - Tutorials: false
Checking if HashSet of Objects Contains an Entry or Not
The following example shows the usage of Java HashSet contains() method to check entries to be present in a HashSet. We've created a HashSet object of Student objects. Then few entries are added using add() method and then set is printed. An existing entry is checked using contains() method and then a non-existing entry is checked and results are printed.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Student> newset = new HashSet <>(); // populate hash set newset.add(new Student(1, "Julie")); newset.add(new Student(2, "Robert")); newset.add(new Student(3, "Adam")); // print the set System.out.println("Hash set: "+ newset); // checking elements in hash set System.out.println("Hash set contains - Julie: "+ newset.contains(new Student(1, "Julie"))); System.out.println("Hash set contains - Jene: "+ newset.contains(new Student(4, "Jene"))); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } @Override public boolean equals(Object obj) { if(obj == null) return false; Student s = (Student)obj; return this.rollNo == s.rollNo && this.name.equalsIgnoreCase(s.name); } @Override public int hashCode() { return rollNo + name.hashCode(); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set: [[ 2, Robert ], [ 1, Julie ], [ 3, Adam ]] Hash set contains - Julie: true Hash set contains - Jene: false
To Continue Learning Please Login