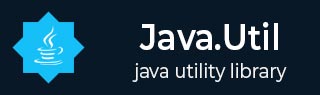
- Java.util Package Classes
- Java.util - Home
- Java.util - ArrayDeque
- Java.util - ArrayList
- Java.util - Arrays
- Java.util - BitSet
- Java.util - Calendar
- Java.util - Collections
- Java.util - Currency
- Java.util - Date
- Java.util - Dictionary
- Java.util - EnumMap
- Java.util - EnumSet
- Java.util - Formatter
- Java.util - GregorianCalendar
- Java.util - HashMap
- Java.util - HashSet
- Java.util - Hashtable
- Java.util - IdentityHashMap
- Java.util - LinkedHashMap
- Java.util - LinkedHashSet
- Java.util - LinkedList
- Java.util - ListResourceBundle
- Java.util - Locale
- Java.util - Observable
- Java.util - PriorityQueue
- Java.util - Properties
- Java.util - PropertyPermission
- Java.util - PropertyResourceBundle
- Java.util - Random
- Java.util - ResourceBundle
- Java.util - ResourceBundle.Control
- Java.util - Scanner
- Java.util - ServiceLoader
- Java.util - SimpleTimeZone
- Java.util - Stack
- Java.util - StringTokenizer
- Java.util - Timer
- Java.util - TimerTask
- Java.util - TimeZone
- Java.util - TreeMap
- Java.util - TreeSet
- Java.util - UUID
- Java.util - Vector
- Java.util - WeakHashMap
- Java.util Package Extras
- Java.util - Interfaces
- Java.util - Exceptions
- Java.util - Enumerations
- Java.util Useful Resources
- Java.util - Useful Resources
- Java.util - Discussion
Java HashSet clone() Method
Description
The Java HashSet clone() method is used to return a shallow copy of this HashSet instance.
Declaration
Following is the declaration for java.util.HashSet.clone() method.
public Object clone()
Parameters
NA
Return Value
The method call returns a shallow copy of this set.
Exception
NA
Cloning a HashSet of Integers Example
The following example shows the usage of Java HashSet clone() method to create a shallow copy of the HashSet. We've created two HashSet objects of Integers. Then few entries are added using add() method one set and then set is printed. Now set is cloned using clone() method and printed.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Integer> newset = new HashSet <>(); HashSet <Integer> newset1 = new HashSet <>(); // populate hash set newset.add(1); newset.add(2); newset.add(3); // checking elements in hash set System.out.println("Hash set values: "+ newset); // clone the set newset1 = (HashSet<Integer>) newset.clone(); // print the set System.out.println("Cloned Hash set values: "+ newset1); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set values: [1, 2, 3] Cloned Hash set values: [1, 2, 3]
Cloning a HashSet of Strings Example
The following example shows the usage of Java HashSet clone() method to create a shallow copy of the HashSet. We've created two HashSet objects of Strings. Then few entries are added using add() method one set and then set is printed. Now set is cloned using clone() method and printed.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <String> newset = new HashSet <>(); HashSet <String> newset1 = new HashSet <>(); // populate hash set newset.add("Learning"); newset.add("Easy"); newset.add("Simply"); // checking elements in hash set System.out.println("Hash set values: "+ newset); // clone the set newset1 = (HashSet<String>) newset.clone(); // print the set System.out.println("Cloned Hash set values: "+ newset1); } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set values: [Learning, Easy, Simply] Cloned Hash set values: [Learning, Easy, Simply]
Cloning a HashSet of Objects Example
The following example shows the usage of Java HashSet clone() method to create a shallow copy of the HashSet. We've created two HashSet objects of Student objects. Then few entries are added using add() method one set and then set is printed. Now set is cloned using clone() method and printed.
package com.tutorialspoint; import java.util.HashSet; public class HashSetDemo { public static void main(String args[]) { // create hash set HashSet <Student> newset = new HashSet <>(); HashSet <Student> newset1 = new HashSet <>(); // populate hash set newset.add(new Student(1, "Julie")); newset.add(new Student(2, "Robert")); newset.add(new Student(3, "Adam")); // checking elements in hash set System.out.println("Hash set values: "+ newset); // clone the set newset1 = (HashSet<Student>) newset.clone(); // print the set System.out.println("Cloned Hash set values: "+ newset1); } } class Student { int rollNo; String name; Student(int rollNo, String name){ this.rollNo = rollNo; this.name = name; } @Override public String toString() { return "[ " + this.rollNo + ", " + this.name + " ]"; } }
Output
Let us compile and run the above program, this will produce the following result.
Hash set values: [[ 1, Julie ], [ 2, Robert ], [ 3, Adam ]] Cloned Hash set values: [[ 2, Robert ], [ 1, Julie ], [ 3, Adam ]]
To Continue Learning Please Login