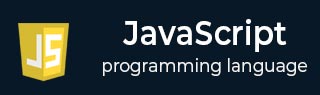
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - WeakMap() Constructor
In JavaScript, the WeakMap is a built-in object that allows us to store key-value pairs in a similar way to a regular Map, but with a few key differences −
Keys − In WeakMap, keys can only be objects, not primitive values like string or numbers.
Size − WeakMaps do not have a size property or forEach() method to get the number of items in a WeakMap or iterate over its elements.
Clear − WeakMaps do not have a clear() method to get remove all the elements from a WeakMap at once. However, we can remove individual elements by deleting their key from the WeakMap using delete() method.
Not enumerable − The keys of a WeakMap are not enumerable, so we cannot use methods like keys(), values(), or entries() to get the keys or values of a WeakMap.
Garbage collection − WeakMap objects are used to store weakly held objects, Which means that the keys and values can be garbage collected if they are no longer referenced anywhere else in your code.
The WeakMap() constructor creates a new WeakMap object. It can take an optional iterable argument, which is an iterable object whose elements are key-value pairs. If provided, the key-value pairs are added to the new WeakMap object.
The WeakMap() constructor can only be constructed with new keyword. If we attempt to invoke it without 'new', throws a TypeError.
Syntax
Following is the syntax of JavaScript WeakMap() constructor −
new WeakMap() new WeakMap(iterable)
Parameters
This constructor accepts an optional parameter. The same is described below −
iterable (optional) − It can be any iterable object that contains array-like objects with two elements: the key and the value. If no iterable argument is provided, an empty WeakMap object is created.
Return Value
The return value of the WeakMap() constructor is a new WeakMap object.
Example 1
In the following example, we are creating an empty WeakMap object using the JavaScript WeakMap() constructor −
<html> <body> <script> const weakMap = new WeakMap(); if(weakMap){ document.write("Weakmap object created successfully...") } </script> </body> </html>
Output
If we execute the above program, an empty WeakMap object will be created.
Example 2
We can also pass an optional iterable object as a parameter to the WeakMap() constructor as shown below −
<html> <body> <script> let key1 = {id: 1}; let key2 = {id: 2}; let key3 = {id: 3}; const weakMap = new WeakMap([ [key1, 'apple'], [key2, 'banana'], [key3, 'cherry'] ]); document.write(weakMap.get(key1) + ", " + weakMap.get(key2) + ", " + weakMap.get(key3)); </script> </body> </html>
Output
The above program returns the values associated with their respective keys.
Example 3
We can also add key-value pairs to a WeakMap object after it has been created using the set() method as shown in the following example −
<html> <body> <script> let weakMap = new WeakMap(); let key1 = {}; let key2 = {}; let key3 = {}; weakMap.set(key1, "apple"); weakMap.set(key2, "banana"); weakMap.set(key3, "cherry"); document.write(weakMap.get(key1) + ", " + weakMap.get(key2) + ", " + weakMap.get(key3)); </script> </body> </html>
Output
The "weakMap" object will contain the provided three key-value pairs as elements. Also, if we execute the program, we can see the "values" associated with their respective keys.
Example 4
We can access the value associated with a key in a WeakMap object using the get() method as shown in the below example −
<html> <body> <script> let weakMap = new WeakMap(); let id1 = {id: 1}; let id2 = {id: 2}; let id3 = {id: 3}; weakMap.set(id1, "apple"); weakMap.set(id2, "banana"); weakMap.set(id3, "cherry"); document.write(weakMap.get(id1), "<br>"); document.write(weakMap.get(id2), "<br>"); document.write(weakMap.get(id3)); </script> </body> </html>
Output
The above program prints the "values" associated with the keys 'id1', 'id2', and 'id3' in the WeakMap object.
Example 5
Here, we are using the delete() method to delete a key-value pair from a WeakMap object −
<html> <body> <script> let weakMap = new WeakMap(); let id1 = {id :1}; let id2 = {id :2}; let id3 = {id :3}; weakMap.set(id1, "apple"); weakMap.set(id2, "banana"); weakMap.set(id3, "cherry"); document.write(weakMap.delete(id1), "<br>"); document.write(weakMap.delete(id2)); </script> </body> </html>
Output
The above program removes the key-value pair associated with the key 'id1' and 'id2' from the WeakMap object.
To Continue Learning Please Login