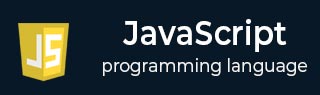
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray with() Method
The JavaScript TypedArray with() method is used change the value of a given index. It returns a new typed array with the element at index replaced with the specified value.
This method throws a 'RangeError' exception, if the index parameter value is either less than or greater than the typed array length.
Syntax
Following is the syntax of JavaScript TypedArray with() method −
arrayInstance.with(index, value)
Parameters
index − The zero-based index at which the value will be will replaced or changed.
value − A value to be insert or assigned at the specified index.
Return value
This method returns a new typed array with the element at specified index replaced with value.
Examples
Example 1
Replace the value of first element (index = 0), with the specified value.
In the following example we are using the JavaScript TypedArray with() method to change (or replace) this typed array [10, 20, 30, 40, 50] element at specified index 0 with the value 10.
<html> <head> <title>JavaScript TypedArray with() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50]); document.write("Typed array: ", T_array); const index = 0; const value = 0; document.write("<br>Index: ", index); document.write("<br>Value: ", value); var new_arr = ([]); //using with() method new_arr = T_array.with(index, value); document.write("<br>New typed array after replacing value at specified index: ", new_arr); </script> </body> </html>
Output
Once the above program is executed, it will returns a new typed array after replacing [0, 20, 30, 40, 50].
Typed array: 10,20,30,40,50 Index: 0 Value: 0 New typed array after replacing value at specified index: 0,20,30,40,50
Example 2
The following is another example of the JavaScript TypedArray with() method. We use this method to change (or replace) the value of this typed array [1, 2, 3, 4, 5, 6, 7] at specified index 3 with the given value 10.
<html> <head> <title>JavaScript TypedArray with() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7] ); document.write("Typed array: ", T_array); const index = 3; const value = 10; document.write("<br>Index: ", index); document.write("<br>Value: ", value); var new_arr = ([]); //using with() method new_arr = T_array.with(index, value); document.write("<br>New typed array after replacing value at specified index: ", new_arr); </script> </body> </html>
Output
The above program returns a new typed array as [1,2,3,10,5,6,7].
Typed array: 1,2,3,4,5,6,7 Index: 3 Value: 10 New typed array after replacing value at specified index: 1,2,3,10,5,6,7
Example 3
If the index parameter value is greater than the typed array length, it will throw a 'RangeError' exception.
<html> <head> <title>JavaScript TypedArray with() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50, 60, 70, 80]); document.write("Typed array: ", T_array); const index = 10; const value = 1; document.write("<br>Index: ", index); document.write("<br>Value: ", value); document.write("<br>Typed array length: ", T_array.length); const new_arr = ([]); try { new_arr = T_array.with(index, value); } catch (error) { document.write("<br>", error); } </script> </body> </html>
Output
After executing the above program, it will throw a 'RangeError' exception.
Typed array: 10,20,30,40,50,60,70,80 Index: 10 Value: 1 Typed array length: 8 RangeError: Invalid typed array index
To Continue Learning Please Login