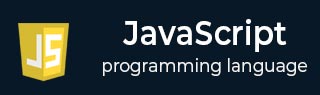
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray toLocaleString() Method
The JavaScript TypedArray toLocaleString() method returns a string representation of the current typed array elements. The typed array elements are converted to strings using this method, and these strings are separated with the specified locale-specific separator such as a comma (","), a symbol ($, ₹), etc.
The specified locale parameter is BCP (best current practice) 47 language tags are standard for the representation of regionalized language.
Syntax
Following is the syntax of JavaScript TypedArray toLocaleString() method −
toLocaleString(locales, options)
Parameters
This method accepts two parameters named 'locales' and 'options', which are described below −
locales − It is a string with a BCP 47 language tag that specifies the language and formatting options.
options − An object is used as an option to change the style, currency, minimum and maximum fraction digits, etc.
Return value
This method returns a string representation of the current typed array elements.
Examples
Example 1
If both locales and options parameters are omitted, it converts the elements of the current typed array [1000, 205, 5993, 4032] to strings considering your system locale and separates the strings with a comma (',').
<html> <head> <title>JavaScript TypedArray toLocaleString() Method</title> </head> <body> <script> const T_array = new Int16Array([1000, 205, 5993, 4032]); document.write("Typed array: ", T_array); //using toLocaleString() method let str = T_array.toLocaleString(); document.write("<br>The string representing typed array elements: ", str); </script> </body> </html>
Output
The above program returns a string representing the typed array element −
Typed array: 1000,205,5993,4032 The string representing typed array elements: 1,000,205,5,993,4,032
Example 2
If we pass only the "locale" parameter to this method, it converts the typed array elements to string based on the specified locale.
The following is another example of the JavaScript TypedArray toLocaleString() method. We use this method to retrieve a string representation of the elements of this typed array [4023, 6123, 30, 146] based on the specified locale "de-DE".
<html> <head> <title>JavaScript TypedArray toLocaleString() Method</title> </head> <body> <script> const T_array = new Int16Array([4023, 6123, 30, 146]); document.write("Typed array: ", T_array); const locale = "de-DE"; document.write("<br>The locale: ", locale); //using toLocaleString() method let str = T_array.toLocaleString(locale); document.write("<br>The string representing typed array elements: ", str); </script> </body> </html>
Output
After executing the above program, it will return a string representing the typed array elements as −
Typed array: 4023,6123,30,146 The locale: de-DE The string representing typed array elements: 4.023,6.123,30,146
Example 3
If we pass both the locale and the options parameters to this method, it will convert the typed array elements to strings based on the specified locale and options (configure the properties like the currency symbol).
In the example below, we use the JavaScript TypedArray toLocaleString() method to retrieve a string representation of the elements of this typed array [5034, 6946, 234, 1003] using the specified country locale "en-In" and the currency symbol "₹".
<html> <head> <title>JavaScript TypedArray toLocaleString() Method</title> </head> <body> <script> const T_array = new Int16Array([5034, 6946, 234, 1003]); document.write("Typed array: ", T_array); const locale = "en-IN"; const options = {style: "currency", currency: "INR"}; document.write("<br>The locale: ", locale); document.write("<br>The options: ", options.style,", ",options.currency); //using toLocaleString() method let str = T_array.toLocaleString(locale, options); document.write("<br>The string representing typed array elements: ", str); </script> </body> </html>
Output
Once the above program is executed, it will display the output as shown below −
Typed array: 5034,6946,234,1003 The locale: en-IN The options: currency, INR The string representing typed array elements: ₹5,034.00,₹6,946.00,₹234.00,₹1,003.00
To Continue Learning Please Login