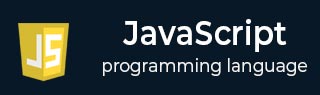
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray subarray() Method
The JavaScript TypedArray subarray() method is used to retrieve a sub-part of this typed array. It does not modify the original typed array but returns a new typed array object. It accepts two parameters that specify the position (index) of elements in the typed array.
Syntax
Following is the syntax of JavaScript TypedArray subarray() method −
subarray(begin, end)
Parameters
This method accepts two optional parameters named 'begin' and 'end', which are described below −
begin − It is the starting position from which the element at begin.
end − It is the end position at which the element at end(not included).
Return value
This method returns a new typed array object.
Examples
Example 1
If you omit both the 'begin' and 'end' parameters, this method returns a new typed array object that includes all the elements of the original typed array. So, if you don’t specify any range, it essentially creates a copy of the entire array.
In the following program, we are using the JavaScript TypedArray subarray() method to retrieve a sub-part of this typed array [1, 2, 3, 4, 5].
<html> <head> <title>JavaScript TypedArray subarray() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5]); document.write("Original typed array: ", T_array); let new_t_array = new Uint8Array([]); new_t_array = T_array.subarray(); document.write("<br>New typed array: ", new_t_array); </script> </body> </html>
Output
The above program returns a new typed array as: [1, 2, 3, 4, 5].
Original typed array: 1,2,3,4,5 New typed array: 1,2,3,4,5
Example 2
If we omit the end parameter and pass only the 'begin' parameter, this method returns a new typed array that starts with the specified begin position till the last element.
In this example, we are using the subarray() method on a typed array to retrieve a sub-part of this typed array [10, 20, 30, 40, 50, 60, 70, 80]. The subarray starts from the specified starting position 2.
<html> <head> <title>JavaScript TypedArray subarray() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50, 60, 70, 80]); document.write("Original typed array: ", T_array); const begin = 2; document.write("<br>Begin at: ", begin); let new_t_array = new Uint8Array([]); new_t_array = T_array.subarray(begin); document.write("<br>New typed array: ", new_t_array); </script> </body> </html>
Output
After executing the above program, it will return a new typed array as: [30, 40, 50, 60, 70, 80].
Original typed array: 10,20,30,40,50,60,70,80 Begin at: 2 New typed array: 30,40,50,60,70,80
Example 3
If we pass both the 'begin' and 'end' parameters to this method, it returns a new typed array that starts with the specified begin position and extends up to, but excludes the end position.
In the following program, we are using the subarray() method on a typed array to retrieve a sub-part of this typed array [2, 4, 6, 8, 10, 12, 14, 16, 18, 20], starting from position 4, and ending just before position 8.
<html> <head> <title>JavaScript TypedArray subarray() Method</title> </head> <body> <script> const even_t_array = new Uint8Array([2, 4, 6, 8, 10, 12, 14, 16, 18, 20]); document.write("Original typed array: ", even_t_array); const begin = 4; const end = 8; document.write("<br>Begin at: ", begin); document.write("<br>End at: ", end); let new_t_array = new Uint8Array([]); new_t_array = even_t_array.subarray(begin, end); document.write("<br>New typed array: ", new_t_array); </script> </body> </html>
Output
Once the above program is executed, it returns a new typed array as −
Original typed array: 2,4,6,8,10,12,14,16,18,20 Begin at: 4 End at: 8 New typed array: 10,12,14,16
To Continue Learning Please Login