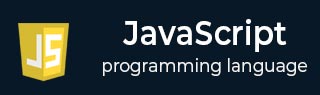
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray slice() Method
The JavaScript TypedArray slice() method returns a copy of the extracted elements from the original typed array into a new typed array object, selected from the start index up to the end, but not including, the end index. The start and end indices represent the positions of the elements in the typed array.
This method does not modify or change the original typed array, but returns a new typed array containing the extracted elements. If we pass a negative value for the start parameter, it will start extracting elements from the end position of the typed array. For example, if start = −1, it will represent the last element.
Syntax
Following is the syntax of JavaScript TypedArray slice() method −
slice(start, end)
Parameters
This method accepts two optional parameters named 'start' and 'end', which are described below −
start (optional) − The zero-based index at which the elements start extracting.
end (optional) − The zero-based index at which the extraction will stop (the slice() method extracts up to, but does not include, that end).
Return value
This method returns a new typed array containing the extracted elements of the original typed array.
Examples
Example 1
If we omit both the 'start' and 'end' parameters, the slice() method begins extracting elements from the zeroth index and continues until the last index of the original typed array.
In the following program, we use the JavaScript slice() method to extract a portion of the original typed array [1, 2, 3, 4, 5, 6, 7, 8] into a new typed array object. Since we haven't specified start and end parameters for this method, it copies all elements from the original array.
<html> <head> <title>JavaScript TypedArray slice() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Original TypedArray: ", T_array); //using the slice() method T_array.slice(); document.write("<br>New TypedArray(after copying):", T_array); </script> </body> </html>
Output
The above program returns a new TypedArray as [1, 2, 3, 4, 5, 6, 7, 8].
Original TypedArray: 1,2,3,4,5,6,7,8 New TypedArray(after copying):1,2,3,4,5,6,7,8
Example 2
If we only pass the start parameter to this method, it extracts elements from the specified start position and continues extracting until the last element in the original typed array.
The following is another example of the JavaScript TypedArray slice() method. In this case, we are trying to extract elements from the original typed array [10, 20, 30, 40, 50], starting at the specified start position 2, and continuing until the last element.
<html> <head> <title>JavaScript TypedArray slice() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50]); document.write("Original TypedArray: ", T_array); const start = 2; document.write("<br>Start(extracting position) value: ", start); //creating new empty typed array let new_t_array = new Uint8Array([]); //using the slice() method new_t_array = T_array.slice(start); document.write("<br>New TypedArray(after copying): ", new_t_array); </script> </body> </html>
Output
After executing the above program, it will display the new typed array as [30, 40, 50].
Original TypedArray: 10,20,30,40,50 Start(extracting position) value: 2 New TypedArray(after copying): 30,40,50
Example 3
If we pass both the 'start' and 'end' parameters to this method, it extracts elements starting from the specified 'start' position and continues extracting up to, but not including, the 'end' position.
In this example, we are using the TypedArray slice() method to extract a copy of a portion of an original typed array [20, 40, 60, 70, 90, 100, 120, 130] from starting specified start position 3, up to the specified end position 6 (not included).
<html> <head> <title>JavaScript TypedArray slice() Method</title> </head> <body> <script> const T_array = new Uint8Array([20, 40, 60, 70, 90, 100, 120, 130]); document.write("Original TypedArray: ", T_array); const start = 3; const end = 6; document.write("<br>Start value: ", start); document.write("<br>End value: ", end); //creating new empty typed array let new_t_array = new Uint8Array([]); //using the slice() method new_t_array = T_array.slice(start, end); document.write("<br>New TypedArray(after copying): ", new_t_array); </script> </body> </html>
Output
Once the above program is executed, it display the new TypedArray as −
Original TypedArray: 20,40,60,70,90,100,120,130 Start value: 3 End value: 6 New TypedArray(after copying): 70,90,100
Example 4
If we pass the start parameter value as -2 to the slice() method, it will extract the last two elements from the typed array [1, 2, 3, 4, 5].
<html> <head> <title>JavaScript TypedArray slice() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5]); document.write("Original TypedArray: ", T_array); const start = -2; document.write("<br>Start value: ", start); //creating new empty typed array let new_t_array = new Uint8Array([]); //using the slice() method new_t_array = T_array.slice(start); document.write("<br>New TypedArray(after copying): ", new_t_array); </script> </body> </html>
Output
The above program returns a new typed array as: [4, 5].
Original TypedArray: 1,2,3,4,5 Start value: -2 New TypedArray(after copying): 4,5
To Continue Learning Please Login