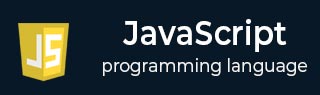
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray set() Method
The JavaScript TypedArray set() method is used to store one or multiple values in the typed array by reading the input values from the specified source array. It returns none(undefined).
Additionally, it accepts an optional parameter named 'targetOffset', which specifies the position in the target array at which the writing begins. If not specified, it starts writing at the 0th index in the target array.
Note − If the targetOffset parameter value is negative or exceeds the target array length, it will throw a "RangeError" exception.
Syntax
Following is the syntax of JavaScript TypedArray set() method −
set(typedarray, targetOffset)
Parameters
This method accepts two parameters named "typedarray" and "targetOffset", which are described below −
typedarray− The source array from which to copy values.
targetOffset (optional)− The index (or position) within the target array at which to begin writing values.
Return value
This method returns None(undefined).
Examples
Example 1
In the following program, we are using the JavaScript TypedArray set() method to store multiple values in the typed array by reading input values from the specified source array [1, 2, 3, 4, 5, 6, 7, 8].
<html> <head> <title>JavaScript TypedArray set() Method</title> </head> <body> <script> const T_array = new Uint16Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Typed array(target array): ", T_array); //using the set() method document.write("<br>The set() method returns: ", T_array.set([0, 0, 0])); </script> </body> </html>
Output
Once the above program is executed, it will return an 'undefined'.
Typed array(target array): 1,2,3,4,5,6,7,8 The set() method returns: undefined
Example 2
If the targetOffset parameter is omitted, then the source array will overwrite values in the target array starting at index 0.
The following is another example of the JavaScript TypedArray set() method. We use this method to store multiple values in this typed array [10, 20, 30, 40, 50] by reading the input values from the specified source array [1, 2, 3] starting at default index 0.
<html> <head> <title>JavaScript TypedArray set() Method</title> </head> <body> <script> const T_array = new Uint16Array([10, 20, 30, 40, 50]); document.write("Typed array(target array): ", T_array); const source_arr = new Uint16Array([1, 2, 3]); document.write("<br>Source array: ", source_arr); //using the set() method T_array.set(source_arr); document.write("<br>Typed array after set: ", T_array); </script> </body> </html>
Output
The above program returns a typed array as [1, 2, 3, 40, 50].
Typed array(target array): 10,20,30,40,50 Source array: 1,2,3 Typed array after set: 1,2,3,40,50
Example 3
If we pass the targetOffset parameter value as 2, it starts storing the values in the target array from the specified source array, beginning at the specified targetOffset value.
In the given example below, we use the JavaScript TypedArray set() method to store the multiple values in the target typed array [0,0,0,0,0,0,0,0] by reading the input values from the specified source array [10,20,30] starting at specified index 2.
<html> <head> <title>JavaScript TypedArray set() Method</title> </head> <body> <script> const T_array = new Uint16Array([0, 0, 0, 0, 0, 0, 0, 0]); document.write("Typed array(target array): ", T_array); const source_arr = new Uint16Array([10, 20, 30]); document.write("<br>Source array: ", source_arr); const targetOffset = 2; document.write("<br>The targetOffset: ", targetOffset); //using the set() method T_array.set(source_arr, targetOffset); document.write("<br>Typed array after set: ", T_array); </script> </body> </html>
Output
After executing the above program, it will display the following output −
Typed array(target array): 0,0,0,0,0,0,0,0 Source array: 10,20,30 The targetOffset: 2 Typed array after set: 0,0,10,20,30,0,0,0
Example 4
If the targetOffset parameter value is passed as −2, since it is a negative value, the set() method throws a "RangeError" exception.
<html> <head> <title>JavaScript TypedArray set() Method</title> </head> <body> <script> const T_array = new Uint16Array([1, 3, 5, 7, 9]); document.write("Typed array(target array): ", T_array); const source_arr = new Uint16Array([10, 20, 30]); document.write("<br>Source array: ", source_arr); const targetOffset = -2; document.write("<br>The targetOffset: ", targetOffset); //using the set() method try { T_array.set(source_arr, targetOffset); document.write("<br>Typed array after set: ", T_array); } catch (error) { document.write("<br>", error); } </script> </body> </html>
Output
Once the above program is executed, it will throw a "RangeError" exception.
Typed array(target array): 1,3,5,7,9 Source array: 10,20,30 The targetOffset: -2 RangeError: offset is out of bounds
To Continue Learning Please Login