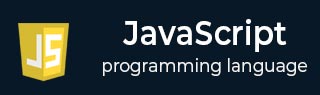
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray of() Method
The JavaScript TypdArray of() method creates a new typed array from a specified variable number of arguments. It returns a typed array instance containing the elements provided as arguments. The arguments must be of the same type as the elements of the resulting typed array.
If we pass the argument value as 'undefined' or 'empty', it will create a new typed array with an element value of 0.
This is a static method, which means it can be called without creating an instance of the TypedArray object (as TypedArray.of()). The TypedArray object can be any of the subclasses of TypedArray, such as Uint8Array, Int16Array, etc.
Syntax
Following is the syntax of JavaScript TypedArray of() method −
TypedArray.of(element1, element2, /*..., */ elementN)
Parameters
This method accepts multiple parameters of the same type namely: 'element1', 'element2', ....'elementN'. The same is described below −
element1,..., elementN − The elements used to create the new typed array.
Return value
This method returns an instance of the new typed array.
Examples
Example 1
If an empty('') string is passed, it will be converted to the number 0 and added to the typed array.
In the following program, we are using the JavaScript TypedArray of() method to create a new typed array with the specified empty('') argument value.
<html> <head> <title>JavaScript TypedArray of() Method</title> </head> <body> <script> //Unit8Array let T_array = Uint8Array.of(''); document.write("The new typed array: ", T_array); document.write("<br>Length of typed array: ", T_array.length); </script> </body> </html>
Output
The above program creates a new typed array as [0].
The new typed array: 0 Length of typed array: 1
Example 2
If we pass multiple arguments of the same type to this method, it will create a new typed array using these argument values.
The following is another example of the JavaScript TypedArray of() method. We use this method to create a new typed array using the specified values '10', '20', '30', '40', and '50'.
<html> <head> <title>JavaScript TypedArray of() Method</title> </head> <body> <script> //Unit16Array let ele1 = '10'; let ele2 = '20'; let ele3 = '30'; let ele4 = '40'; let ele5 = '50'; document.write("Element1 = ", ele1); document.write("<br>Element2 = ", ele2); document.write("<br>Element3 = ", ele3); document.write("<br>Element4 = ", ele4); document.write("<br>Element5 = ", ele5); let T_array = Uint16Array.of(ele1, ele2, ele3, ele4, ele5); document.write("<br>The new typed array: ", T_array); document.write("<br>Length of typed array: ", T_array.length); </script> </body> </html>
Output
Once the above program is executed, it will return an instance of the new typed array as −
Element1 = 10 Element2 = 20 Element3 = 30 Element4 = 40 Element5 = 50 The new typed array: 10,20,30,40,50 Length of typed array: 5
Example 3
If we pass the 'undefined' as an argument to the of() method, it will create a new typed array with the value 0.
In the example below, we use the typed array of() method to create a new typed array with the specified argument value 'undefined'.
<html> <head> <title>JavaScript TypedArray of() Method</title> </head> <body> <script> //Unit8Array let T_array = Uint8Array.of(undefined); document.write("The new typed array: ", T_array); document.write("<br>Length of typed array: ", T_array.length); </script> </body> </html>
Output
After executing the above program, it creates a new typed array with the element value 0.
The new typed array: 0 Length of typed array: 1
Example 4
The of() method will throw a 'TypeError' exception if this value of the method is not a valid typed array constructor. For example, if you try to call the method on a number, a string, or an object, you will get a TypeError.
<html> <head> <title>JavaScript TypedArray of() Method</title> </head> <body> <script> try { let T_array = "Hello".of(1, 2, 3); document.write("The new typed array: ", T_array); document.write("<br>Length of typed array: ", T_array.length); } catch (error) { document.write(error); } </script> </body> </html>
Output
The above program throws a 'TypeError' exception −
TypeError: "Hello".of is not a function
To Continue Learning Please Login