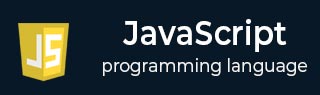
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray lastIndexof() Method
The JavaScript TypedArray lastIndexOf() method searches for a specified searchElement in a typed array from right to left. It returns the index of the last occurrence of the searchElement and -1 if not found.
Suppose the specified searchElement is present only once in the typed array, then this method returns the index starting from the zeroth index. If the searchElement is present more than once, it will give the first priority to the last element.
Syntax
Following is the syntax of JavaScript TypedArraylastIndexof() method −
lastIndexOf(searchElement, fromIndex)
Parameters
This method takes in two parameters, namely 'searchElement' and 'fromIndex'. The parameters are described below −
searchElement − The element that needs to be searched in the typed array.
fromIndex − The zero-based index indicates the position to start searching from the end.
Return value
This method returns the last index of the searchElement in a typed array. If the search element is not present, it returns −1.
Examples
Example 1
If the fromIndex parameter is omitted and only the searchElement parameter is passed, the method returns the last index of the specified searchElement from the end of the TypedArray.
In the following example, we are using the JavaScript TypedArray method lastIndexOf() to retrieve the last index of the specified searchElement 30 in the typed array [10, 20, 30, 40, 30, 50, 60].
<html> <head> <title>JavaScript TypedArray lastIndexof() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 30, 40, 50, 60]); document.write("Original typed array: ", T_array); const serachElement = 30; document.write("<br>Search element: ", serachElement); document.write("<br>The element ", serachElement, " found at the last index ", T_array.lastIndexOf(serachElement)); </script> </body> </html>
Output
The above program returns the index of the last occurrence of the element 30 as 3.
Original typed array: 10,20,30,30,40,50,60 Search element: 30 The element 30 found at the last index 3
Example 2
If we pass both 'searchElement' and 'fromIndex' parameters to this method, it returns the last index of the element starting at the specified fromIndex.
The following is another example of the JavaScript TypedArray lastIndexOf() method. We are using this method to retrieve the last index of the specified element 6, starting the search from index 5, in this typed array: [1, 2, 4, 6, 8, 9, 6, 11].
<html> <head> <title>JavaScript TypedArray lastIndexof() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2 , 4, 6, 8, 9, 6, 11]); document.write("Original typed array: ", T_array); const serachElement = 6; const fromIndex = 5; document.write("<br>Search element: ", serachElement); document.write("<br>From index: ", fromIndex); document.write("<br>The element ", serachElement, " found at the last index ", T_array.lastIndexOf(serachElement, fromIndex)); </script> </body> </html>
Output
After executing the program, the index of the last occurrence of element 6 is returned as 3.
Original typed array: 1,2,4,6,8,9,6,11 Search element: 6 From index: 5 The element 6 found at the last index 3
Example 3
If the specified searchElement is not found in this typed array, it returns -1.
In this example, we are using the typed array lastIndexOf() method on a typed array to retrieve the last index of the searchElement 11 in the given typed array [1, 3, 5, 7, 13].
<html> <head> <title>JavaScript TypedArray lastIndexof() Method</title> </head> <body> <script> const T_array = new Uint8Array( [1, 3, 5, 7, 13]); document.write("Original typed array: ", T_array); const serachElement = 6; document.write("<br>Search element: ", serachElement); document.write("<br>The element ", serachElement, " found at the last index ", T_array.lastIndexOf(serachElement)); </script> </body> </html>
Output
Once the above program is executed, it will return -1.
Original typed array: 1,3,5,7,13 Search element: 6 The element 6 found at the last index -1
To Continue Learning Please Login