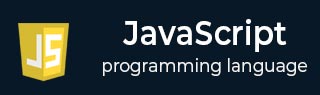
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray keys() Method
The JavaScript TypedArray keys() method returns an array iterator object that contains the keys for each index of the typed array. A key in JavaScript is a unique identifier used to access values in an object.
Syntax
Following is the syntax of JavaScript TypedArray keys() method −
keys()
Parameters
It does not accept any parameters.
Return value
This method returns a new array iterator object.
Examples
Example 1
In the following program, we are using the JavaScript TypedArray keys() method to retrieve an array iterator object of this typed array [10, 20, 30, 40, 50].
<html> <head> <title>JavaScript TypedArray keys() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50]); document.write("The typed arrays is: ", T_array); document.write("<br>The T_array.keys() method returns: ", T_array.keys()); </script> </body> </html>
Output
The above program return '[object Array Iterator]' in the output.
The typed arrays is: 10,20,30,40,50 The T_array.keys() method returns: [object Array Iterator]
Example 2
The following is another example of the JavaScript TypedArray keys(). We use this method to retrieve an array iterator object containing keys of each index of this typed array [1, 2, 3, 4, 5, 6, 7, 8].
<html> <head> <title>JavaScript TypedArray keys() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50]); document.write("The typed arrays is: ", T_array); document.write("<br>The T_array.keys() method returns: ", T_array.keys()); const arrayKeys = T_array.keys(); document.write("<br>The typed array keys and respected values are: <br>") for(const n of arrayKeys){ document.write("Key = ", n, " , Value = ", T_array[n], "<br>"); } </script> </body> </html>
Output
After executing the above program, it will display keys on each index along with values as −
The typed arrays is: 10,20,30,40,50 The T_array.keys() method returns: [object Array Iterator] The typed array keys and respected values are: Key = 0 , Value = 10 Key = 1 , Value = 20 Key = 2 , Value = 30 Key = 3 , Value = 40 Key = 4 , Value = 50
Example 3
In this program, we use the keys() method to retrieve an array iterator object containing the keys of each index in the typed array [1, 2, 3, 4, 5]. Then, we store all keys in a variable and perform an alternative iteration.
<html> <head> <title>JavaScript TypedArray keys() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5]); document.write("The typed arrays is: ", T_array); const allKeys = T_array.keys(); document.write("<br>"); document.write("Key1 = ", allKeys.next().value, "<br>"); document.write("Key2 = ", allKeys.next().value, "<br>"); document.write("Key3 = ", allKeys.next().value, "<br>"); document.write("Key4 = ", allKeys.next().value, "<br>"); document.write("Key5 = ", allKeys.next().value); </script> </body> </html>
Output
Once the above program is executed, it will return the keys of each index as −
The typed arrays is: 1,2,3,4,5 Key1 = 0 Key2 = 1 Key3 = 2 Key4 = 3 Key5 = 4
To Continue Learning Please Login