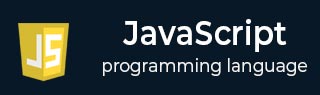
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray join() Method
The JavaScript TypedArray join() method is used to create and return a string by concatenating typed array elements and separating them with a comma (the default separator) or the specified separator string. If the current typed array contains only one element, the element will be returned as is without using the separator.
If the current typed array is empty (or arr.length is 0), an empty string is returned.
Syntax
Following is the syntax of JavaScript TypedArray join() method −
join(separator)
Parameters
This method accepts a parameter named 'separator', which is described below −
separator (optional) − The string separates each element of the typed array while concatenating.
Return value
This method returns a string concatenated with all typed array elements.
Examples
Example 1
If the separator parameter is omitted, it concatenates the typed array elements, separated by commas.
In the following program, we are using the JavaScript TypedArray join() method to concatenates this typed array elements [1, 2, 3, 4, 5, 6, 7, 8] separated with commas.
<html> <head> <title>JavaScript TypedArray join() Method</title> </head> <body> <script> const T_array = new Uint16Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Typed array: ", T_array); //using join() method let new_str = ""; new_str = T_array.join(); document.write("<br>New concatenated string: ", new_str); </script> </body> </html>
Output
The above returns the concatenated string with comma separated as −
Typed array: 1,2,3,4,5,6,7,8 New concatenated string: 1,2,3,4,5,6,7,8
Example 2
If we pass the separator parameter value as "−", it will concatenate the typed array elements and separate them with the specified separator.
The following is another example of the JavaScript TypedArray join() method. We use this method to concatenate this typed array elements [10, 20, 30, 40, 50, 60, 70, 80, 90, 100] and separate them with the specified separator string "−".
<html> <head> <title>JavaScript TypedArray join() Method</title> </head> <body> <script> const T_array = new Uint16Array([10, 20, 30, 40, 50, 60, 70, 80, 90, 100]); document.write("Typed array: ", T_array); const separator_str = "-"; document.write("<br>Separator: ", separator_str); //using join() method let new_str = ""; new_str = T_array.join(separator_str); document.write("<br>New concatenated string: ", new_str); </script> </body> </html>
Output
After executing the above program, it will return a new concatenated string separated by "−".
Typed array: 10,20,30,40,50,60,70,80,90,100 Separator: - New concatenated string: 10-20-30-40-50-60-70-80-90-100
Example 3
If the current typed array has only one element "new Uint16Array([100])", then the same element will be returned without using a separator.
<html> <head> <title>JavaScript TypedArray join() Method</title> </head> <body> <script> const T_array = new Uint16Array([100]); document.write("Typed array: ", T_array); const separator_str = "-#-"; document.write("<br>Separator: ", separator_str); //using join() method let new_str = ""; new_str = T_array.join(separator_str); document.write("<br>New concatenated string: ", new_str); </script> </body> </html>
Output
Once the above program is executed, it will return the concatenated string without using a separator.
Typed array: 100 Separator: -#- New concatenated string: 100
To Continue Learning Please Login