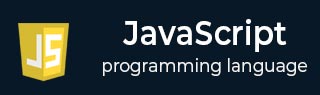
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray indexof() Method
The JavaScript TypedArray indexOf() method is used to retrieve the first index at which the specified element is found or located. If the specified element is repeated twice in the typed array, the method will prioritize the first occurrence and return its index, not the second one.
If we specify the fromIndex parameter value to this method, it starts searching for the element from the specified fromIndex. If the element is not found between the fromIndex and the last index of the typed array, it returns −1, even if the element is present in the typed array.
Syntax
Following is the syntax of JavaScript TypedArray indexOf() method −
indexOf(searchElement, fromIndex)
Parameters
This method accepts two parameters named 'searchElement' and 'fromIndex'. The same as described below −
searchElement − The element to be searched.
fromIndex − The zero-based index(position) at which to start searching.
Return value
This method returns the first index of the searchElement.
Examples
Example 1
If we omit the fromIndex parameter and pass only the searchElement parameter to this method, it searches for this element in the entire typed array and returns the first index where the searchElement is found.
In the following program, we are using the JavaScript TypedArray indexOf() method to retrieve the first index of the specified element 2 in this typed array [1, 2, 3, 4, 5, 6, 7, 8].
<html> <head> <title>JavaScript TypedArray indexof() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Original TypedArray: ", T_array); const searchElement = 3; document.write("<br>searchElement: ", searchElement); document.write("<br>The element ", searchElement, " is found at position ", T_array.indexOf(searchElement)); </script> </body> </html>
Output
The above program returns the first index as '2'.
Original TypedArray: 1,2,3,4,5,6,7,8 searchElement: 3 The element 3 is found at position 2
Example 2
If we pass both searchElement and fromIndex parameters to this method, it starts searching for the specified searchElement at the specified fromIndex position.
In this example of the JavaScript TypedArray indexOf() method, we are trying to retrieve the first index of the specified element 60, starting the search from the specified fromIndex position 4 in this typed array: [10, 20, 30, 40, 50, 60, 70, 80, 60].
<html> <head> <title>JavaScript TypedArray indexof() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50, 60, 70, 80, 60]); document.write("Original TypedArray: ", T_array); const searchElement = 60; const fromIndex = 4; document.write("<br>searchElement: ", searchElement); document.write("<br>fromIndex: ", fromIndex); document.write("<br>The element ", searchElement, " is found at position ", T_array.indexOf(searchElement, fromIndex)); </script> </body> </html>
Output
After executing the above program, it will return the first index as 5.
Original TypedArray: 10,20,30,40,50,60,70,80,60 searchElement: 60 fromIndex: 4 The element 60 is found at position 5
Example 3
If the specified element is not present in the typed array, the indexOf() method returns -1.
In this example, we use the indexOf() method on a typed array to retrieve the first index of the element 50. However, this element is not present between the fromIndex 4 and the last index of the typed array [10, 20, 50, 30, 90, 70].
<html> <head> <title>JavaScript TypedArray indexof() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 50, 30, 90, 70]); document.write("Original TypedArray: ", T_array); const searchElement = 50; const fromIndex = 4; document.write("<br>searchElement: ", searchElement); document.write("<br>fromIndex: ", fromIndex); document.write("<br>The element ", searchElement, " is found at position ", T_array.indexOf(searchElement, fromIndex)); </script> </body> </html>
Output
Once the above program is executed, it will return -1.
Original TypedArray: 10,20,50,30,90,70 searchElement: 50 fromIndex: 4 The element 50 is found at position -1
To Continue Learning Please Login