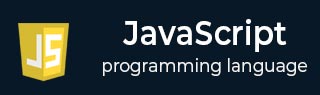
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray includes() Method
The JavaScript TypedArray includes() method is used to determine whether a searchElement value is present in the entire typed array or within the range from fromIndex (the starting position) to the end of the typed array. This method returns a boolean value 'true' if the specified searchElement is found within the typed array. If the searchElement is not found, it returns 'false'.
Syntax
Following is the syntax of JavaScript TypedArray includes() method −
includes(searchElement, fromIndex)
Parameters
This method accepts two parameters named 'searchElement' and 'fromIndex', which are described below −
searchElement − The element to be searched in the typed array.
fromIndex − The zero-based index at which start searching the searchElement.
Return value
This method returns 'true' if searchElement is found in the typed array; 'false' otherwise.
Examples
Example 1
If the specified searchElement is not found within the typed array, it will return 'false'.
In the following example, we are using the JavaScript TypedArray includes() method to determine whether the specified searchElement 20 is found in this typed array [10, 40, 30, 50, 80, 100].
<html> <head> <title>JavaScript TypedArray includes() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 40, 30, 50, 80, 100]); document.write("The typed array elements are: ", T_array); const searchElement = 20; document.write("<br>The elenent to be searched: ", searchElement); //using the includes() method document.write("<br>Does element ", searchElement, " is found in typed array ? ", T_array.includes(searchElement)); </script> </body> </html>
Output
The above programs returns 'false'.
The typed array elements are: 10,40,30,50,80,100 The elenent to be searched: 20 Does element 20 is found in typed array ? false
Example 2
If the specified searchElement is found within the typed array, it will return 'true'.
The following is another example of the JavaScript TypedArray includes() method. We use this method to determine whether the specified searchElement 4 is found in this typed array [1, 2, 3, 4, 5, 6, 7, 8].
<html> <head> <title>JavaScript TypedArray includes() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("The typed array elements are: ", T_array); const searchElement = 4; document.write("<br>The elenent to be searched: ", searchElement); //using the includes() method document.write("<br>Does element ", searchElement, " is found in typed array ? ", T_array.includes(searchElement)); </script> </body> </html>
Output
After executing the above program, it returns 'true'.
The typed array elements are: 1,2,3,4,5,6,7,8 The elenent to be searched: 4 Does element 4 is found in typed array ? true
Example 3
If both 'searchElement' and 'fromIndex' parameters are passed to this method, it starts searching for the 'searchElement' from the specified 'fromIndex' in the typed array.
In this program, we use the includes() method to determine whether the specified searchElement 2 is found between the starting position 4, and till the end of this typed array [1, 2, 3, 5, 7, 9, 6, 8, 11, 15].
<html> <head> <title>JavaScript TypedArray includes() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 5, 7, 9, 6, 8, 11, 15]); document.write("The typed array elements are: ", T_array); const searchElement = 2; const fromIndex = 3; document.write("<br>The elenent to be searched: ", searchElement); document.write("<br>The starting position: ", fromIndex); //using the includes() method document.write("<br>Does element ", searchElement, " is found between start index ", fromIndex, " and till the end of typed array ? ", T_array.includes(searchElement, fromIndex)); </script> </body> </html>
Output
Once the above program is executed, it will return 'false'.
The typed array elements are: 1,2,3,5,7,9,6,8,11,15 The elenent to be searched: 2 The starting position: 3 Does element 2 is found between start index 3 and till the end of typed array ? false
To Continue Learning Please Login