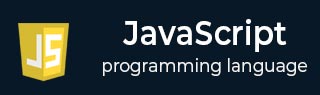
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray forEach() Method
The JavaScript TypedArray forEach() method executes a provided function once for each element in a typed array and returns None(undefined).
Here are some additional points you should know about the 'forEach()' method −
The forEach() method operates on a TypedArray (such as Uint8Array, Int16Array, etc.).
It accepts a testing function as a parameter.
The testing function is executed for each element in the TypedArray.
Syntax
Following is the syntax of JavaScript TypedArray forEach() method −
forEach(callbackFn, thisArg)
Parameters
This method accepts two parameters named 'callbackFn' and 'thisArg', which are described below −
callbackFn − This parameter is a testing function that will be executed for each element in the TypedArray.
This function takes three parameters named 'element', 'index', and 'array'. Here's the description for each parameter −
element − Represents the current element being processed in the TypedArray.
index − Indicates the index (position) of the current element within the TypedArray.
array − Refers to the entire TypedArray.
thisArg (optional) − This is an optional parameter that allows you to specify the value of this within the callbackFn.
Return value
This method returns None(undefined).
Examples
Example 1
In the following program, we use the JavaScript TypedArray forEach() method to execute the provided function once for each element. We create an arrow function that iterates through each element of this typed array [10, 20, 30, −40, −50, 60] and passes it as an argument to this method.
<html> <head> <title>JavaScript TypedArray forEach() Method</title> </head> <body> <script> const T_array = new Int16Array([10, 20, 30, -40, -50, 60]); document.write("Typed array: ", T_array); //using forEach() method document.write("<br>The typed array elements: ") T_array.forEach((element)=>{ document.write(element); }); </script> </body> </html>
Output
The above program returns the each element of typed array as −
Typed array: 10,20,30,-40,-50,60 The typed array elements: 102030-40-5060
Example 2
The following is another example of the JavaScript TypedArray forEach() method. We use this method to execute the provided function named display() once for each typed array element. We pass this function as an argument to this method, and the function checks the index and values of the typed array element and passes it as an argument to this method.
<html> <head> <title>JavaScript TypedArray forEach() Method</title> </head> <body> <script> function display(element, index, array){ document.write("<br>a[",index,"]", " = ", element); } const T_array = new Int16Array([10, 20, 30, -40, -50, 60]); document.write("Typed array: ", T_array); //using forEach() method T_array.forEach(display); </script> </body> </html>
Output
After executing the above program, it returns indexes and values of typed array elements.
Typed array: 10,20,30,-40,-50,60 a[0] = 10 a[1] = 20 a[2] = 30 a[3] = -40 a[4] = -50 a[5] = 60
Example 3
As we had discussed earlier, the forEach() method doesn't return a value. Let's verify it with a suitable example −
<html> <head> <title>JavaScript TypedArray forEach() Method</title> </head> <body> <script> function display(element, index, array){ return element > 0; } const T_array = new Int16Array([10, 20, 30, -40, -50, 60]); document.write("Typed array: ", T_array); //using forEach() method document.write("<br>The forEach() method returns: ", T_array.forEach(display)); </script> </body> </html>
Output
The above program returns "undefined".
Typed array: 10,20,30,-40,-50,60 The forEach() method returns: undefined
To Continue Learning Please Login