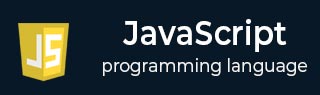
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray findLastIndex() Method
The JavaScript TypedArray findLastIndex() method iterates the typed array in reverse order, which means that the last element of the typed array will be considered as first element and vice versa. The method returns the index of the first element (from last) that satisfies the provided testing function. If none of the elements of the typed array satisfy the testing function, the method will return '−1'.
Here are some additional points you should know about the 'findLastIndex()' method −
The findLastIndex() method operates on a TypedArray (such as Uint8Array, Int16Array, etc.).
It accepts a testing function as a parameter.
The testing function is executed for each element in the TypedArray.
If an element satisfies the condition specified by the testing function (it returns a true value).
If no element satisfies the testing function −1 is returned.
Syntax
Following is the syntax of JavaScript TypedArray findLastIndex() method −
findLastIndex(callbackFn, thisArg)
Parameters
This method accepts two parameters named 'callbackFn' and 'thisArg', which are described below −
callbackFn − This parameter is a testing function that will be executed for each element in the TypedArray.
This function takes three parameters named 'element', 'index', and 'array'. Here's the description for each parameter −
element − Represents the current element being processed in the TypedArray.
index − Indicates the index (position) of the current element within the TypedArray.
array − Refers to the entire TypedArray.
thisArg (optional) − This is an optional parameter that allows you to specify the value of this within the callbackFn.
Return value
This method returns the index of the last element that satisfies the testing function. If no element satisfies the testing function, '−1' is returned.
Examples
Example 1
If the element satisfies the testing function, it will return the index of the last element in the typed array.
In the following example, we are using the JavaScript TypedArray findLastIndex() method to retrieve the index of the last element that satisfied the providing testing function. We create a testing function named isEven() checks for even numbers, and passes it as an argument to this method.
<html> <head> <title>JavaScript TypedArray findLastIndex() Method</title> </head> <body> <script> function isEven(element, index, array){ return element %2 == 0; } const T_array = new Uint8Array([10, 20, 30, 40, 50, 60, 70, 80, 81]); document.write("Typed array: ", T_array); //calling function document.write("<br>The index of the last even number is: ", T_array.findLastIndex(isEven)); </script> </body> </html>
Output
The above program returns the index of the last even number as 7.
Typed array: 10,20,30,40,50,60,70,80,81 The index of the last even number is: 7
Example 2
Find the index of the last leap year in TypedArray.
In the given example below, we create a function named isLeapYear() that checks for leap year in this typed array [2002, 2004, 2005, 2006, 2007, 2014], and we pass this function as an argument to findLastIndex() method to retrieve the last leap year that satisfies the testing function.
<html> <head> <title>JavaScript TypedArray findLastIndex() Method</title> </head> <body> <script> function isLeapYear(element, index, arrar){ return ((element % 4 == 0) && (element % 400 == 0 || element % 100 !=0)); } const T_array = new Int16Array([2002, 2004, 2005, 2006, 2007, 2012]); document.write("Typed array: ", T_array); //calling function document.write("<br>The index of the last leap year in typed array is: ", T_array.findLastIndex(isLeapYear)); </script> </body> </html>
Output
Once the above program is executed, it will return the index of the last leap year in typed array as −
Typed array: 2002,2004,2005,2006,2007,2012 The index of the last leap year in typed array is: 5
Example 3
If no element satisfies the testing function, the findLastIndex() method will return '-1'.
The following is another example of the JavaScript TypedArray findLastIndex() method. We create a function named isNegative() that checks for the negative numbers, and passes it as an argument to this method to retrieve the index of the last element that satisfies the testing function.
<html> <head> <title>JavaScript TypedArray findLastIndex() Method</title> </head> <body> <script> function isNegative(element, index, array){ return element < 0; } const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Typed array: ", T_array); //calling function document.write("<br>The index of last negative element: ", T_array.findLastIndex(isNegative)); </script> </body> </html>
Output
After executing the above program, it will return '-1'.
Typed array: 1,2,3,4,5,6,7,8 The index of last negative element: -1
To Continue Learning Please Login