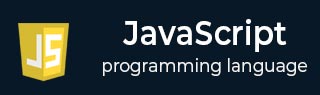
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray find() Method
The JavaScript TypeArray find() method returns the value of the first element in the current typed array that satisfies the provided testing function. If no values are satisfied or no value is passed to the testing function, undefined is returned.
Here's the some additional points about find() method −
The find() method operates on a TypedArray (such as Uint8Array, Int16Array, etc.).
It accepts a testing function as a parameter.
The testing function is executed for each element in the TypedArray.
If an element satisfies the condition specified by the testing function (returns a true value), that element is returned as the result.
If no elements pass to the testing function, undefined is returned.
Syntax
Following is the syntax of JavaScript TypedArray find() method −
find(callbackFn, thisArg)
Parameters
This method accepts two parameters named 'callbackFn' and 'thisArg', which are described below −
callbackFn − This parameter is a testing function that will be executed for each element in the TypedArray.
This function takes three parameters named 'element', 'index', and 'array'. Here's the description for each parameter −
element − Represents the current element being processed in the TypedArray.
index − Indicates the index (position) of the current element within the TypedArray.
array − Refers to the entire TypedArray.
thisArg (optional) − This is an optional parameter that allows you to specify the value of this within the callbackFn.
Return value
This method returns the first element is TypedArray that satisfied the provided test function, 'undefined' otherwise.
Examples
Example 1
In the following example, we use the JavaScript TypedArray find() method to search for the first even number in the original TypedArray [1, 2, 3, 4, 5, 6, 7, 8]. We create a testing function named isEven() that checks if a number is even, and then pass this function as an argument to the find() method.
<html> <head> <title>JavaScript TypedArray find() Method</title> </head> <body> <script> function isEven(element, index, array){ return element %2 == 0; } const T_array = new Int8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Orizinal TypedArray: ", T_array); document.write("<br>First even number in TypedArray is: "); document.write(T_array.find(isEven)); </script> </body> </html>
Output
The above program returns the first even number as '2' in the TypedArray [1, 2, 3, ,4 ,5 ,6 ,7, 8]
Orizinal TypedArray: 1,2,3,4,5,6,7,8 First even number in TypedArray is: 2
Example 2
The following is another example of the JavaScript TypedArray find(). We use this method to search for the first negative number in this typed array [1, 2, -1, 0, 1, 2, -2, -3]. We create a testing function named isNegative() that checks if a number is negative and then pass this function as an argument to the find() method.
<html> <head> <title>JavaScript TypedArray find() Method</title> </head> <body> <script> function isNegative(element, index, array){ return element < 0; } const T_array = new Int8Array([1, 2, -1, 0, 1, 2, -2, -3]); document.write("Orizinal TypedArray: ", T_array); document.write("<br>First negative number in TypedArray is: "); document.write(T_array.find(isNegative)); </script> </body> </html>
Output
After executing the above program, it returns first negative number as -1 in the TypedArray [1, 2, -1, 0, 1, 2, -2, -3].
Orizinal TypedArray: 1,2,-1,0,1,2,-2,-3 First negative number in TypedArray is: -1
Example 3
If no value satisfies the testing function, it will return 'undefined'.
In this program, we create a function called isPrime() that checks whether a number is prime. We use this function as an argument to the find() function to retrieve the first prime number in the TypedArray [4, 6, 8, 9]. However, since no value satisfies the isPrime() function, the find() method returns undefined.
<html> <head> <title>JavaScript TypedArray find() Method</title> </head> <body> <script> function isPrime(element, index, array){ let begin = 2; while(begin <= Math.sqrt(element)){ if(element % begin++ < 1){ return false; } } return element > 1; } const T_array = new Int8Array([4, 6, 8, 9]); document.write("Orizinal TypedArray: ", T_array); document.write("<br>First negative number in TypedArray is: "); document.write(T_array.find(isPrime)); </script> </body> </html>
Output
Once the above program is executed, it will return 'undefined'.
Orizinal TypedArray: 4,6,8,9 First negative number in TypedArray is: undefined
To Continue Learning Please Login