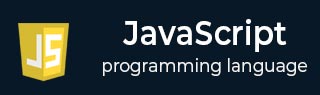
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray fill() Method
The JavaScript TypedArray fill() method is used to modify all the elements in this typed array within a specified range of indices (from start to end). We need to provide an element (referred to as value) to replace the existing values.
The start and end parameters are optional, which means if you haven't passed them to this method, it modifies the entire array by filling it with the specified value. This method modifies the original array and does not return a new typed array.
Syntax
Following is the syntax of JavaScript TypedArray fill() method −
fill(value, start, end)
Parameters
This method accepts three parameters named 'value', 'start', and 'end'. The explanation of these parameters is as follows −
value − The value to to be filled in the typed array.
start (optional)− The zero-based index starting position at which starts filling the value.
end (optional)− The zero-based index end position at which to stop filling.
Return value
This method returns the modified typed array, that is filled with the specified value.
Examples
Example 1
If we omit the 'start' and 'end' parameters and only pass the 'value' parameter to this method, it changes and fills the entire typed array with the specified value.
In the following program, we are using the JavaScript TypedArray fill() method to change and fill the entire typed array [0, 0, 0, 0, 0] with the specified value 1.
<html> <head> <title>JavaScript TypedArray fill() Method</title> </head> <body> <script> const T_array = new Uint8Array([0, 0, 0, 0, 0]); document.write("The typed array before filled: ", T_array); const value = 1; document.write("<br>The value to be filled: ", value) //using fill() method T_array.fill(value); document.write("<br>The typed array after filled: ", T_array); </script> </body> </html>
Output
The above program returns a modified typed array filled with value 1 as [1, 1, 1, 1, 1].
The typed array before filled: 0,0,0,0,0 The value to be filled: 1 The typed array after filled: 1,1,1,1,1
Example 2
If we pass the 'value' and 'start' parameters, this method will fill the typed array at the specified start position with the specified value.
The following is another example of the JavaScript TypedArray fill() method. We use this method to change this typed array [1, 2, 3, 4, 5, 6, 7, 8], and fill it with the specified value 0, at the starting position 2.
<html> <head> <title>JavaScript TypedArray fill() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("The typed array before filled: ", T_array); const value = 0; const start = 2; document.write("<br>The value to be filled: ", value); document.write("<br>The starting position: ", start); //using fill() method T_array.fill(value, start); document.write("<br>The typed array after filled: ", T_array); </script> </body> </html>
Output
After executing the above program, it will return a modified typed array filled with value 0 starting at position 2 as −
The typed array before filled: 1,2,3,4,5,6,7,8 The value to be filled: 0 The starting position: 2 The typed array after filled: 1,2,0,0,0,0,0,0
Example 3
If all three parameters 'value', 'start', and 'end' are passed, the fill() method changes the typed array by filling the specified value within the range of [start, end].
In this program, we are using the fill() method to change this typed array [10, 20, 30, 40, 50, 60, 70, 80] by filling the specified value 100, with the specified range of [2, 5].
<html> <head> <title>JavaScript TypedArray fill() Method</title> </head> <body> <script> const T_array = new Uint8Array([10, 20, 30, 40, 50, 60, 70, 80]); document.write("The typed array before filled: ", T_array); const value = 100; const start = 2; const end = 5; document.write("<br>The value to be filled: ", value); document.write("<br>The starting position: ", start); document.write("<br>The end position: ", end); //using fill() method T_array.fill(value, start, end); document.write("<br>The typed array after filled: ", T_array); </script> </body> </html>
Output
Once the above program is executed, it will return a modified typed array as [10,20,100,100,100,60,70,80].
The typed array before filled: 10,20,30,40,50,60,70,80 The value to be filled: 100 The starting position: 2 The end position: 5 The typed array after filled: 10,20,100,100,100,60,70,80
To Continue Learning Please Login