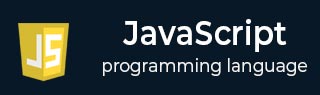
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - TypedArray copyWithin() Method
The JavaScript TypedArray copyWithin() method copies elements within the same TypedArray to another location and returns the modified TypedArray without changing its original length. It accepts three parameters, one of named is the target.
If the target parameter value is negative, it will start inserting an element at the end of the typed array. For example, if target = −1, the value will be inserted at the end of the current typed array.
In JavaScript, TypedArrays are array like objects that provide a mechanism for reading and writing raw binary data in memory buffers.
Syntax
Following is the syntax of JavaScript TypedArray copyWithin() method −
copyWithin(target, start, end)
Parameters
This method accepts three parameters named 'target', 'start', and 'end', which are described below −
target − The Zero-based index (position) at which the elements start inserting.
start − The zero-based index at which the elements start copying.
end (optional) −The Zero-based index at which end to copying the elements. The method copies up to the end but excludes it.
Return value
This method returns a modified TypedArray without changing the length of the original TypedArray.
Examples
Example 1
If we pass only the target and start parameters to this method, it starts inserting elements at the specified target position (which is 3), and it begins copying elements from the original TypedArray starting at the specified start position (which is 0) until the length of the copied elements is equivalent to the original TypedArray ([1, 2, 3, 4, 5, 6, 7, 8]).
<html> <head> <title>JavaScript TypedArray copyWithin() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Original TypedArray: ", T_array); const target = 3; const start = 0; document.write("<br>Target(start insert) position at: ", target); document.write("<br>Start copying position at: ", start); T_array.copyWithin(target, start); document.write("<br>Modified TypedArray: ", T_array); </script> </body> </html>
Output
The above program returns modified typed array as [1,2,3,1,2,3,4,5].
Original TypedArray: 1,2,3,4,5,6,7,8 Target(start insert) position at: 3 Start copying position at: 0 Modified TypedArray: 1,2,3,1,2,3,4,5
Example 2
If we pass all three parameters (target, start, and end) to this method, it starts inserting elements at the specified target position (which is 4), and it begins copying elements from the original TypedArray starting at the specified start position (which is 1) up to, but excluding, the specified end position (which is 3). The remaining elements remain unchanged.
<html> <head> <title>JavaScript TypedArray copyWithin() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 0, 0, 0, 0, 0]); document.write("Original TypedArray: ", T_array); const target = 4; const start = 1; const end = 3; document.write("<br>Target(start insert) position at: ", target); document.write("<br>Start copying position at: ", start); document.write("<br>End(stop copying) position at: ", end); T_array.copyWithin(target, start, end); document.write("<br>Modified TypedArray: ", T_array); </script> </body> </html>
Output
After executing the above program, it returns a modified typed array as [1,2,3,0,2,3,0,0].
Original TypedArray: 1,2,3,0,0,0,0,0 Target(start insert) position at: 4 Start copying position at: 1 End(stop copying) position at: 3 Modified TypedArray: 1,2,3,0,2,3,0,0
Example 3
If the target parameter value is negative (-1), it inserts the element at the end of this TypedArray [1, 2, 3, 4, 5, 6, 7, 8].
<html> <head> <title>JavaScript TypedArray copyWithin() Method</title> </head> <body> <script> const T_array = new Uint8Array([1, 2, 3, 4, 5, 6, 7, 8]); document.write("Original TypedArray: ", T_array); const target = -1; const start = 1; const end = 3; document.write("<br>Target(start insert) position at: ", target); document.write("<br>Start copying position at: ", start); document.write("<br>End(stop copying) position at: ", end); T_array.copyWithin(target, start, end); document.write("<br>Modified TypedArray: ", T_array); </script> </body> </html>
Output
Once the above program is executed, it returns a modified TypedArray as −
Original TypedArray: 1,2,3,4,5,6,7,8 Target(start insert) position at: -1 Start copying position at: 1 End(stop copying) position at: 3 Modified TypedArray: 1,2,3,4,5,6,7,2
To Continue Learning Please Login