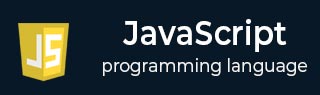
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Array splice() Method
The JavaScript Array.splice() method is used to modify the contents of an array by removing or replacing existing elements or adding new elements. It accepts parameters as the starting index, the no.of elements to be removed, and elements to add(optional). Then it returns an array containing the removed elements. For example, array.splice(3, 5) removes five elements starting from index 3.
This method does not create a new array for result instead, it overwrites the original array.
Syntax
Following is the syntax of JavaScript Array splice() method −
array.splice(start, deleteCount, item1, item2, ..., itemN)
Parameters
This method accepts three parameters. The same is described below −
- start − The index at to add or remove elements. If negative, it counts from the end of the array.
- deleteCount − This specifies the number elements to be removed in the array. If it is 0, no elements are removed.
- item1, ..., itemN − The elements to add to the array, beginning from the start index.
Return value
This method returns an array containing the deleted elements. If no elements are deleted, it returns an empty array.
Examples
Example 1
In the following example, we are using the JavaScript Array splice() method to remove all the array elements, starting from the index postion 2.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; const result = animals.splice(2); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
After executing the program, all the elements from index position 2 are removed.
Output
Lion,Cheetah
Example 2
If the 'deleteCount' parameter of the splice() method is provided as 0, no element will be removed from the animals array.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; const result = animals.splice(2, 0); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
Output
Lion,Cheetah,Tiger,Elephant,Dinosaur
Example 3
The following program removes 0 (zero) elements before index position 2, and inserts the new element “Elephant”.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger"]; const result = animals.splice(2, 0, "Elephant"); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
Output
As we can see the output, the element “Elephant” is inserted at index postion 2 without removing any exising element.
Lion,Cheetah,Elephant,Tiger
Example 4
The following program removes 0 (zero) elements before index position 2, and inserts two new elements i.e. “Elephant” and “Dinosaur”.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger"]; const result = animals.splice(2, 0, "Elephant", "Dinosaur"); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
Output
As we can see the output, the elements “Elephant” and “Dinosaur” is inserted at index postion 2 without removing any exising element.
Lion,Cheetah,Elephant,Dinosaur,Tiger
Example 5
In the following example, we are removing 2 elements, starting from the index position 2.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; const result = animals.splice(2, 2); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
After executing the program, the splice() method removed “Tiger” and “Dinosaur” from the array.
Output
Lion,Cheetah,Dinosaur
Example 6
Here, we are removing 1 element at index position 2, and inserting a new element “Elephant”.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger"]; const result = animals.splice(2, 1, "Elephant"); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
After executing the program, the element “tiger” will be removed and “elephant” will be inserted in that index.
Output
Lion,Cheetah,Elephant
Example 7
Here, we are removing 1 element at index position 2, and inserting two new elements “Elephant” and “Dinosaur”.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger"]; const result = animals.splice(2, 1, "Elephant", "Dinosaur"); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
After executing the program, the element “tiger” will be removed and the elements “elephant” and “Dinosaur” will be inserted.
Output
Lion,Cheetah,Elephant,Dinosaur
Example 8
In the following example, we are removing 1 element from index -2 (counts from end of the array).
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; const result = animals.splice(-2, 1); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
After executing the program, the element “Elephant” will be removed.
Output
Lion,Cheetah,Tiger,Dinosaur
Example 9
Here, we are removing 1 element from index -2 (counts from end of the array), and inserting two new elements “Elephant” and “Dinosaur”.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger"]; const result = animals.splice(-2, 1, "Elephant", "Dinosaur"); document.getElementById("demo").innerHTML = animals; </script> </body> </html>
After executing the program, the element “Cheetah” will be removed and “Elephant” and “Dinosaur” will be removed.
Output
Lion,Elephant,Dinosaur,Tiger
To Continue Learning Please Login