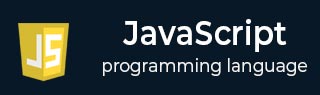
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Array slice() Method
In JavaScript, the Array.slice() method is used to select a portion of elements from an array and return a new array with those selected elements. It accepts two parameters: "start" index and the "end" index. It selects from a given "start", up to a given "end". If we do not provide the end index, the method extracts to the end of the array.
This method does not modify the existing array instead; it returns a new array containing the shallow copy of the selected elements.
Syntax
Following is the syntax of JavaScript Array slice() method −
array.slice(start, end);
Parameters
This method accepts two parameters. The same is described below −
- start − This is the start position in the array from which we want to select elements. By default, this value is 0.
- end − This is the position in the array before which we want to stop selecting elements. Default value is the last element.
Return value
This method returns a new array containing the extracted elements. It does not modify the original array.
Examples
Example 1
In the following example, we are passing "2" as the start index to the JavaScript Array slice() method.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; let result = animals.slice(2); document.getElementById("demo").innerHTML = result; </script> </body> </html>
After executing the above program, the slice() method selects and returns all the elements from index position 2 to the end of the array.
Output
Tiger,Elephant,Dinosaur
Example 2
Here, we are selecting and returning the elements from the element at index 2 till the element at index 4 −
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; let result = animals.slice(2, 4); document.getElementById("demo").innerHTML = result; </script> </body> </html>
Output
Tiger,Elephant
Example 3
Here, we are passing a negative value “-2” as an argument to the start index of slice() method. So, the method starts selecting the elements from the end of the array.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; let result = animals.slice(-2); document.getElementById("demo").innerHTML = result; </script> </body> </html>
Output
Elephant,Dinosaur
Example 4
In this example, we are passing “-4” as start postion and “−1” as end position. So, it selects and returns elements from the fourth-to-last to the second-to-last in the array.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; let result = animals.slice(-4, -1); document.getElementById("demo").innerHTML = result; </script> </body> </html>
Output
Cheetah,Tiger,Elephant
Example 5
If no arguments are passed, the slice() method returns the result array contains the same elements as the original animals array.
<html> <body> <p id="demo"></p> <script> const animals = ["Lion", "Cheetah", "Tiger", "Elephant", "Dinosaur"]; let result = animals.slice(); document.getElementById("demo").innerHTML = result; </script> </body> </html>
Output
As we can see the output, result array contains same elements as animals array.
Lion,Cheetah,Tiger,Elephant,Dinosaur
To Continue Learning Please Login