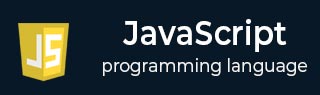
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript - Array .reduce() Method
In JavaScript, the Array.reduce() method is used to manipulate array. This method executes a reducer function on each element of the array (from left to right) and returns a 'single value' as a result.
It accepts an optional parameter named 'initialValue'. If we do not pass this parameter to the method, it will consider the arr[0] value as the initial value. Additionally, it will execute the callback function on the passed initialValue parameter.
This method does not execute the reducer function for empty array elements. In addition to that, it does not modify the original array.
Note − If the current array is empty or doesn't contain any initialValue, this method will throw a 'TypeError' exception.
Syntax
Following is the syntax of JavaScript Array.reduce() method −
reduce(callbackFn(accumulator, currentValue, currentIndex, array), initialValue)
Parameters
This method accepts two parameters. The same is described below −
- callbackFn − This is the function to execute on each element in the array. This function takes four arguments:
- accumulator − This is the initialValue, or the previously returned value of the function.
- currentValue − This is the current element being processed in the array. If the initialValue is specified, its value will be arr[0], if not it's value will be arr[1].
- currentIndex (optional) − This is the index of the current element being processed in the array.
- array (optional)− This is the array on which the reduce() method is called.
- initialValue (optional) − The value to which the accumulator parameter is initialized when the first time the callback function is called.
Return value
This method returns the single value that is the result after reducing the array.
Examples
Example 1
In the following example, we are using the JavaScript Array.reduce() method to sum all the elements present in the provided array.
<html> <body> <script> const numbers = [10, 20, 30, 40, 50]; const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0); document.write(sum); </script> </body> </html>
The accumulator starts at 0, and for each element in the array, it adds the current element to the accumulator. The final result of the accumulator (150) is the sum of all the elements.
Output
150
Example 2
In this example, we are calculating the product of all the elements in the specified array −
<html> <body> <script> const numbers = [10, 20, 30, 40, 50]; const product = numbers.reduce((accumulator, currentValue) => accumulator * currentValue, 1); document.write(product); </script> </body> </html>
The accumulator starts at 1, and for each element in the array, it multiplies the current element by the accumulator. The final result of the accumulator (12000000) is the product of all the elements.
Output
12000000
Example 3
In the below example, we are flattening an array of arrays (nesetedArray) into a single, one dimensional array (flattenedArray) −
<html> <body> <script> const nestedArray = [[1, 2], [3, 4], [5, 6]]; const flattenedArray = nestedArray.reduce((accumulator, currentValue) => accumulator.concat(currentValue), []); document.write(flattenedArray); </script> </body> </html>
Output
1,2,3,4,5,6
Example 4
If the current array does not contain any element(no initial value available), the reduce() method will throw a "TypeError" exception −
<html> <body> <script> const numbers = []; try { numbers.reduce((accumulator, currentValue) => accumulator * currentValue); } catch (error) { document.write(error); } </script> </body> </html>
Output
TypeError: Reduce of empty array with no initial value
To Continue Learning Please Login