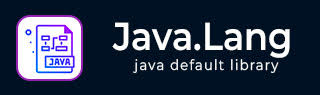
- Java.lang Package classes
- Java.lang - Home
- Java.lang - Boolean
- Java.lang - Byte
- Java.lang - Character
- Java.lang - Character.Subset
- Java.lang - Character.UnicodeBlock
- Java.lang - Class
- Java.lang - ClassLoader
- Java.lang - Compiler
- Java.lang - Double
- Java.lang - Enum
- Java.lang - Float
- Java.lang - InheritableThreadLocal
- Java.lang - Integer
- Java.lang - Long
- Java.lang - Math
- Java.lang - Number
- Java.lang - Object
- Java.lang - Package
- Java.lang - Process
- Java.lang - ProcessBuilder
- Java.lang - Runtime
- Java.lang - RuntimePermission
- Java.lang - SecurityManager
- Java.lang - Short
- Java.lang - StackTraceElement
- Java.lang - StrictMath
- Java.lang - String
- Java.lang - StringBuffer
- Java.lang - StringBuilder
- Java.lang - System
- Java.lang - Thread
- Java.lang - ThreadGroup
- Java.lang - ThreadLocal
- Java.lang - Throwable
- Java.lang - Void
- Java.lang Package extras
- Java.lang - Interfaces
- Java.lang - Errors
- Java.lang - Exceptions
- Java.lang Package Useful Resources
- Java.lang - Useful Resources
- Java.lang - Discussion
Java Class cast() Method
Description
The Java Class cast() method casts an object to the class or interface represented by this Class object.
Declaration
Following is the declaration for java.lang.Class.cast() method
public T cast(Object obj)
Parameters
obj − This is the object to be cast.
Return Value
This method returns the object after casting, or null if obj is null.
Exception
ClassCastException − if the object is not null and is not assignable to the type T.
Casting an Object to a Class Example
The following example shows the usage of java.lang.Class.cast() method. In this program, we've created an instance of ClassDemo and then using getClass() method, the class of the instance is retrieved and is printed. We've two classes A and B where B extends A. Now instance of A and B are created and then using cast() method, instance of B is cast to A.
package com.tutorialspoint; class A { public static void show() { System.out.println("Class A show() function"); } } class B extends A { public static void show() { System.out.println("Class B show() function"); } } public class ClassDemo { public static void main(String[] args) { ClassDemo cls = new ClassDemo(); Class c = cls.getClass(); System.out.println(c); Object obj = new A(); B b1 = new B(); b1.show(); // casts object Object a = A.class.cast(b1); System.out.println(obj.getClass()); System.out.println(b1.getClass()); System.out.println(a.getClass()); } }
Output
Let us compile and run the above program, this will produce the following result −
class com.tutorialspoint.ClassDemo Class B show() function class com.tutorialspoint.A class com.tutorialspoint.B class com.tutorialspoint.B
Facing Exceptio while Casting an Object to a Class Example
The following example shows the usage of java.lang.Class.cast() method. In this program, we've created an instance of ClassDemo and then using getClass() method, the class of the instance is retrieved and is printed. We've two classes A and B. Now instance of A and B are created and then using cast() method, instance of B is cast to A. As B is not compatible to A, an exception is raised.
package com.tutorialspoint; class A { public static void show() { System.out.println("Class A show() function"); } } class B { public static void show() { System.out.println("Class B show() function"); } } public class ClassDemo { public static void main(String[] args) { ClassDemo cls = new ClassDemo(); Class c = cls.getClass(); System.out.println(c); Object obj = new A(); B b1 = new B(); b1.show(); // casts object Object a = A.class.cast(b1); System.out.println(obj.getClass()); System.out.println(b1.getClass()); System.out.println(a.getClass()); } }
Output
Let us compile and run the above program, this will produce the following result −
class com.tutorialspoint.ClassDemo Class B show() function Exception in thread "main" java.lang.ClassCastException: Cannot cast com.tutorialspoint.B to com.tutorialspoint.A at java.base/java.lang.Class.cast(Class.java:4067) at com.tutorialspoint.ClassDemo.main(ClassDemo.java:28)
To Continue Learning Please Login